As a WordPress web developer focused on speed and SEO, I’m always looking for ways to optimize websites without bloating them with plugins. One of the best ways to improve load times is to convert images to WebP format automatically, which offers smaller file sizes without sacrificing quality.
This blog post is a follow-up to my previous article on increase page load speed in WordPress. If you haven’t read it yet, be sure to check it out for an introduction to WordPress speed optimization.
TL;DR Go straight to the code snippet ➡️
Is WebP better than JPG / PNG?
First thing first—why should we even bother about WebP? Well, if performance is important for your site (trust me, it should), then using WebP instead of traditional formats like JPG or PNG can make a huge difference to your WordPress website loading time. Here are few reasons why:
- Modern browser support: Almost all major browsers support WebP these days. No more worrying about compatibility issues.
- Smaller file size: WebP files are often 30-50% smaller than their JPEG/PNG counterparts (sometimes more! depending on the images) without losing quality. This means faster loading times.
- Better SEO performance: Google loves fast websites. Faster pages rank better in search results. So by optimizing your images, you’re indirectly boosting your SEO game too!
- Google recommends it: If you ever check your website’s speed with PageSpeed Insights which is provided by Google, you’ll notice that it will recommend you to serve the image in modern formats like WebP.
In my previous project where I need to rebuild a client website, converting the images alone already improve the website speed so much.
Convert images to WebP snippet
This code snippet was originally by Mark Harris. I modified it so it will add a -1 suffix if you upload the same image twice. I also removed the .gif extension since I honestly think GIFs shouldn’t be converted – this preserves their animation and prevents potential animation issues.
<?php
/**
* Convert Uploaded Images to WebP Format
*
* This snippet converts uploaded images (JPEG, PNG, GIF) to WebP format
* automatically in WordPress. Ideal for use in a theme's functions.php file,
* or with plugins like Code Snippets or WPCodeBox.
*
* @package WordPress_Custom_Functions
* @author Mark Harris
* @link www.christchurchwebsolutions.co.uk
*
* Modification:
* - Added a counter to the filename for duplicate uploads and remove .gif
*
* @author Adit MB
* @link https://webdivo.com
*
* Usage Instructions:
* - Add this snippet to your theme's functions.php file, or add it as a new
* snippet in Code Snippets or WPCodeBox.
* - The snippet hooks into WordPress's image upload process and converts
* uploaded images to the WebP format.
*
* Optional Configuration:
* - By default, the original image file is deleted after conversion to WebP.
* If you prefer to keep the original image file, simply comment out or remove
* the line '@unlink( $file_path );' in the wpturbo_handle_upload_convert_to_webp function.
* This will preserve the original uploaded image file alongside the WebP version.
*/
add_filter( 'wp_handle_upload', 'wpturbo_handle_upload_convert_to_webp' );
function wpturbo_handle_upload_convert_to_webp( $upload ) {
if ( $upload['type'] == 'image/jpeg' || $upload['type'] == 'image/png' ) {
$file_path = $upload['file'];
// Check if ImageMagick or GD is available
if ( extension_loaded( 'imagick' ) || extension_loaded( 'gd' ) ) {
$image_editor = wp_get_image_editor( $file_path );
if ( ! is_wp_error( $image_editor ) ) {
$file_info = pathinfo( $file_path );
$dirname = $file_info['dirname'];
$filename = $file_info['filename'];
$extension = '.webp';
// Create a new file path for the WebP image
$new_file_path = $dirname . '/' . $filename . $extension;
$counter = 1;
// Check for file conflicts and generate a unique filename if needed
while ( file_exists( $new_file_path ) ) {
$new_file_path = $dirname . '/' . $filename . '-' . $counter . $extension;
$counter++;
}
// Attempt to save the image in WebP format
$saved_image = $image_editor->save( $new_file_path, 'image/webp' );
if ( ! is_wp_error( $saved_image ) && file_exists( $saved_image['path'] ) ) {
// Success: replace the uploaded image with the WebP image
$upload['file'] = $saved_image['path'];
$upload['url'] = str_replace( basename( $upload['url'] ), basename( $saved_image['path'] ), $upload['url'] );
$upload['type'] = 'image/webp';
// Optionally remove the original image
@unlink( $file_path );
}
}
}
}
return $upload;
}
How to use the snippet?
- Make sure your hosting enabled the GD or Imagick extentions (most do, but just to be safe).
- Copy the code in your theme’s
function.php
or use a code snippet plugin, I personally use WPCodeBox for it. - Remove the
<?php
if you already have it at the beginning of yourfunctions.php
or code snippet plugin. - Make sure you enabled it in your code snippet plugin.
That’s it! enabling this snippet will automatically convert your JPGs or PNGs to WebP. This image format conversion will ensure quicker page loading and reduced file sizes in WordPress. The downside is this will applies to new image uploads only.
This is an example of the snippet in action. I uploaded two identical images—one with the snippet activated and one without. You can see the difference for yourself! By the way, the image in this example also serves as the featured image for this blog post.
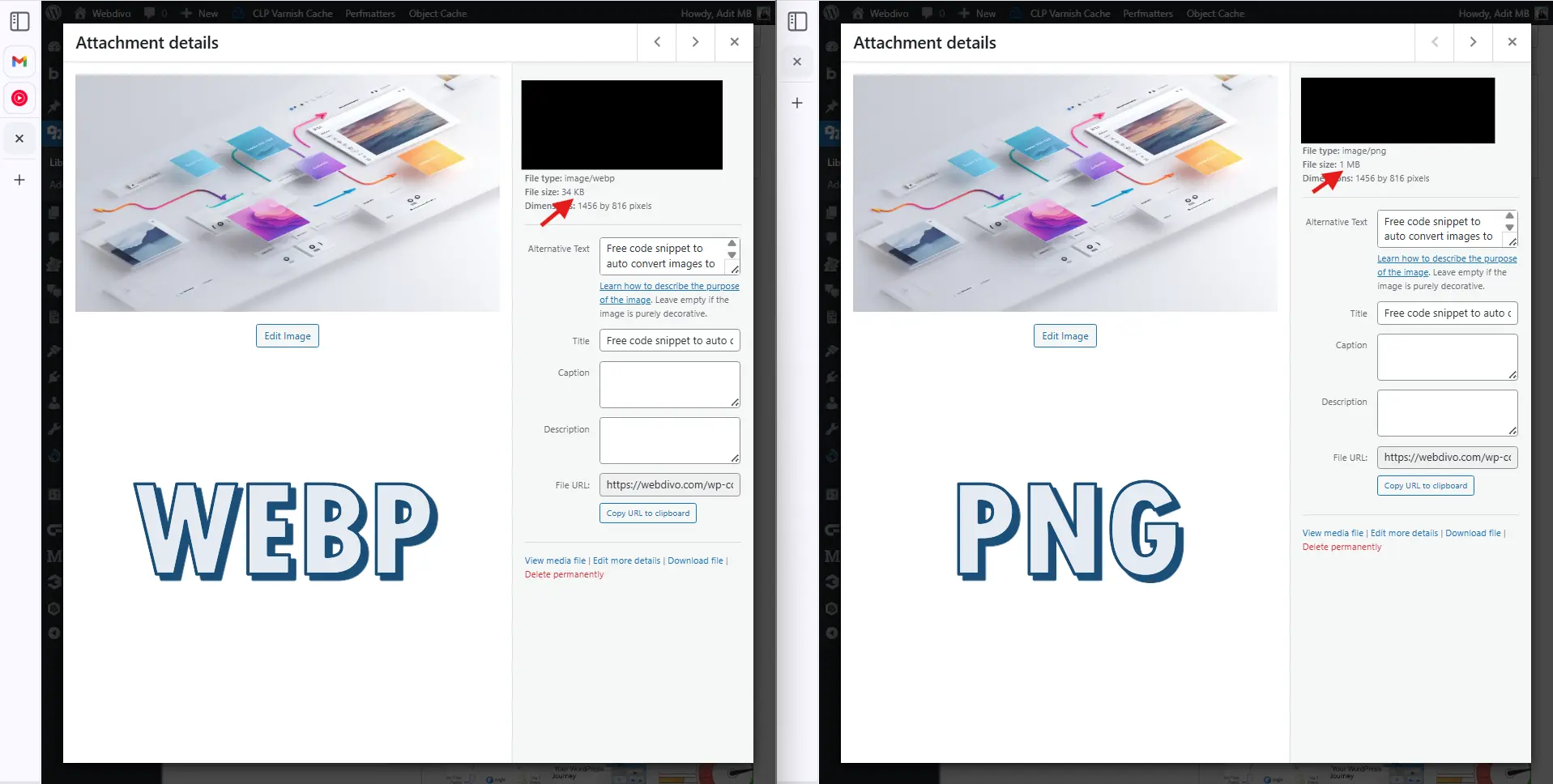
⚠️ Optional: Keep the original image
By default this snippet will remove the original file which is great in my opinion, but if you needed to keep the original image file, all you need to do is to add //
or #
before this: @unlink( $file_path );
on line 67 (or just remove it). It won’t show in WordPress media, but the original image is there in your /wp-content/uploads/
Why WebP is still safer than AVIF
AVIF (AV1 Image File Format) is a promising image format with better compression rates than WebP, but there are still some reasons why WebP remains the safer and more reliable choice for many web developers:
- Wider browser support: AVIF has made significant strides in browser compatibility, with support in major browsers like Chrome, Firefox, Edge, and Safari (since version 16.4). However, there are still some variations in support across different platforms and older browser versions.
- Better compatibility with existing tools: WebP has been around longer than AVIF and is integrated into most image optimization plugins and CMS platforms, including WordPress.
- Image quality vs. file size: AVIF generally offers better compression rates than WebP, meaning smaller file sizes for equivalent quality. However, the quality differences between WebP and AVIF may not always be noticeable to the average user, and AVIF’s encoding can sometimes result in artifacts, especially when aggressively compressed.
While AVIF is a great option for image optimization in certain contexts, WebP remains a safer, more widely supported, and practical solution for most WordPress developers. It strikes a strong balance between image quality, file size, and compatibility, making it the ideal choice for ensuring a fast, visually appealing, and well-optimized website.
Boost your WordPress site speed with WebP conversion
Converting images to WebP in WordPress is a smart move to optimize website speed, improve user experience, and boost SEO performance. I always use this code snippet whenever I start developing a client’s project for Webdivo.
Remember, every millisecond counts in web performance, especially in mobile, and optimizing your images could be the next step toward delivering a faster, more efficient website. So, get started with the code snippet I’ve shared and watch your website’s performance rocketed 🚀