Customizing visibility in Bricks Builder is straightforward, especially when you want specific content to show only on mobile or desktop devices. Using a simple PHP snippet, you can set conditions based on the user’s device type.
Why use visibility conditions?
Device-specific visibility ensures that your content is tailored for different screen sizes, improving user experience. You can always opt for display: none
, but while it hides the content visually, it doesn’t mean the content isn’t rendered in the DOM.
By doing this, in theory, you will have a better mobile performance when creating a WP website.
The snippet
Here’s the PHP code you’ll use. Add this to your child theme’s functions.php
file or use a plugin for custom snippets:
/**
* Snippet Name: Device-Based Visibility
* @author Adit MB
* @link https://webdivo.com
* @version 1.0.1
* Description: Adds a visibility condition in Bricks Builder based on whether
* the user is on a mobile or desktop device using wp_is_mobile().
*
* Usage:
* Add this snippet to your theme's `functions.php` file or a custom code snippet plugin.
*/
if (!defined('ABSPATH')) {
exit;
}
class Device_Based_Visibility {
public function __construct() {
add_action('init', array($this, 'init'));
}
public function init() {
// Add the new visibility condition to Bricks Builder
add_filter('bricks/conditions/options', array($this, 'add_device_visibility_condition'));
// Check the condition result
add_filter('bricks/conditions/result', array($this, 'check_device_visibility_condition'), 10, 3);
}
public function add_device_visibility_condition($options) {
$options[] = [
'key' => 'device_visibility',
'label' => esc_html__('Device', 'bricks'),
'group' => 'other',
'compare' => [
'type' => 'select',
'options' => [
'=' => esc_html__('Is', 'bricks'),
],
],
'value' => [
'type' => 'select',
'options' => [
'mobile' => esc_html__('Mobile', 'bricks'),
'desktop' => esc_html__('Desktop', 'bricks'),
],
],
];
return $options;
}
public function check_device_visibility_condition($result, $condition_key, $condition) {
// Only handle the custom 'device_visibility' condition
if ($condition_key !== 'device_visibility') {
return $result;
}
// Determine if the current device is mobile or desktop
$current_device = wp_is_mobile() ? 'mobile' : 'desktop';
// Get the selected condition values from Bricks
$compare = isset($condition['compare']) ? $condition['compare'] : '=';
$value = isset($condition['value']) ? $condition['value'] : '';
// Compare the current device with the selected value
if ($compare === '=') {
return $current_device === $value;
} elseif ($compare === '!=') {
return $current_device !== $value;
}
return $result;
}
}
new Device_Based_Visibility();
How it works
- Add a new conditions on the item. You will see a new conditions named “Device”.
- Set the conditions whether the item will be displayed if Device is Desktop / Mobile or the other way around.
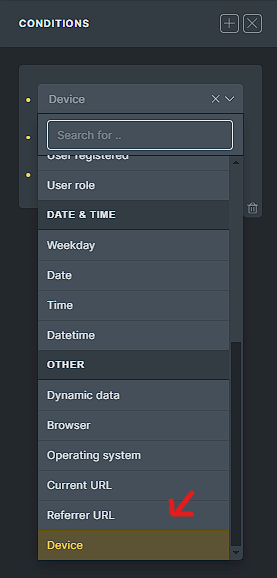
Example
I will give example; in this picture below, I use display: none
on a description wrapper’s div and hide it on tablet’s breakpoint and below, it’s still visible if you inspect:

Now I use the conditional snippet on the .wd-title__desc-wrap so the div and the circular title inside it won’t be rendered in mobile (you can inspect it in this Webdivo’s homepage):

Note:
This snippet is utilizing wp_is_mobile
and to my understanding, if you’re using a cache plugin or CDN and don’t separate the cache for desktop and mobile, it won’t work properly. But if you do separate them, it will work.
For more info about wp_is_mobile
you can visit this link.